In this tutorial of Selenium Framework Mastery series, we will learn multiple ways – How to wait for page load in Selenium with Java? One most common ways is to go with Implicit wait method, and another way is handle web page load is to use Dynamic wait method (FluentWait
or WebDriverWait
).
Let’s dive deep into it –
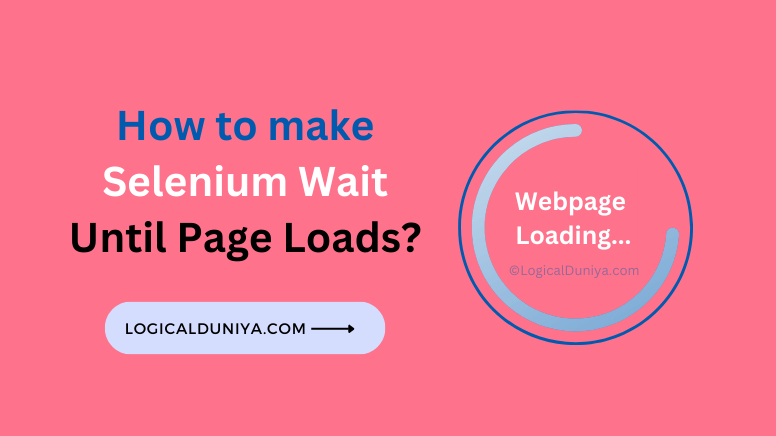
There are multiple solutions to handle web page waiting situation. Selenium provides various ways to wait for a web page to fully load before interacting with its elements. Waiting is essential to ensure that the page’s content, including JavaScript-generated elements, is ready for interaction. Here are a few common techniques to wait for a web page to load using Selenium WebDriver in Java.
Issue : Wait for Page Load in Selenium – Java ?
Using below robust solutions to wait for page load in Selenium, we can achieve our primary goal which is to ensure that the web application is in the desired state when executing Selenium commands and avoiding flakiness in our test results. Let’s see, instead of hard wait such as Thread.sleep(..)
solution, what other solutions do we have and which one is preferred to use in industry –
[Solution – 1] Implicit Wait
An Implicit Wait tells the WebDriver
to wait for a certain amount of time before throwing an exception if an element is not immediately present. Remember one thing before implementing Implicit Wait – This wait is applied globally to all elements.
Code Example :
WebDriver myDriver = new ChromeDriver();
myDriver.manage()
.timeouts()
.implicitlyWait(java.time.Duration.ofSeconds(20));
...
...
myDriver.get("https://abcdwebsite.com/login");
// If the below button is not found immediately, The WebDriver will wait till 20 seconds..
WebElement myLoginButton = myDriver.findElement(By.id("login-btn"));
Code Explanation – Step by Step :
If you set the implicit wait of the WebDriver
, then call the findElement(..)
method on an element you expect to be on the loaded page. Then the WebDriver
will poll for that element until it finds the element or reaches the time out value.
As per the above solution code snippet, as the above 2 statements would be executed, whenever your script launched a web page URL, and tries to search for the login button web element, it would wait till 20 until the web page get loaded completely and it founds that WebElement
.
If it does not find within 20 seconds, then only it will throw the ElementNotFoundException
.
[Solution – 2] Explicit Wait
Explicit waits are more dynamic and customisable in nature.
To use Explicit Wait in your Selenium – Java test scripts, import the following packages into the script-
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
When you implement the Explicit Wait commands, the WebDriver is instructed to wait until a certain condition occurs before proceeding with executing the further code.
It is super important to set the Explicit Wait, specially in cases where there are certain elements that most of the time take more time to load in a web application.
To wait for page load in Selenium, Explicit wait is much more preferred solution than the previous one – implicit wait. Because, If you set an implicit wait command for let’s say : X seconds, then the browser will wait for the same time frame : X seconds, before loading every web element. This causes an unnecessary slowness during the execution of your test script. That’s why, it is always recommended to use Explicit Waits over Implicit Wait.
Code Example :
WebDriverWait theExplicitWait = new WebDriverWait( theDriver, 20);
WebElement theElement = theExplicitWait.until(
ExpectedConditions.presenceOfElementLocated( By.id("login-btn") )
);
Code Explanation – Step by Step :
The above Selenium code snippet uses the WebDriverWait
class along with the ExpectedConditions
class to implement explicit waiting for Page Load, Element Searching or for given condition in Selenium.
Explicit waiting is a technique where the script waits for a specific condition to be met before proceeding with any further actions such as searching for element Or throwing exceptions. This is super useful specially when you want to wait for a specific element to become available immediately after the page load.
Here are more details of this solution of ‘Wait for page load in Selenium – Java‘, do check below points –
1. WebDriverWait theExplicitWait = new WebDriverWait(theDriver, 20);
:
WebDriverWait
is a class provided by Selenium, that allows you to wait for a specific condition to be satisfied.theDriver
is the instance of WebDriver that you’ve initialized for browser automation.20
is the maximum amount of time (in seconds) we have given, that the script will wait for the expected condition to be met.
2. WebElement theElement = theExplicitWait.until(ExpectedConditions.presenceOfElementLocated(By.id("login-btn")));
:
WebElement
is an interface representing a web page element that you can interact with.theElement
is the variable that will store the reference to the web element that meets the expected condition.theExplicitWait.until(...)
waits until the provided condition is satisfied.ExpectedConditions.presenceOfElementLocated(By.id("login-btn"))
is the expected condition. In this case, it waits for an element with the givenid
attribute("login-btn")
to be present in the DOM.
In short, our above code example simply initializes an explicit wait with a maximum timeout of 20 seconds. If the web element (whose id
attribute = “login-btn
” ) is not loaded immediately due to slow Page Load or any other reason, then it waits for that Web Element (with the id
attribute “login-btn
“) to be present in the DOM. Once that element is found, it is assigned to the theElement
variable for further interaction.
This technique ensures that the script waits only as long as necessary for the desired element to be present, making the automation more reliable and preventing unnecessary waits. And, this is how we are handling the slow Page Load scenario.
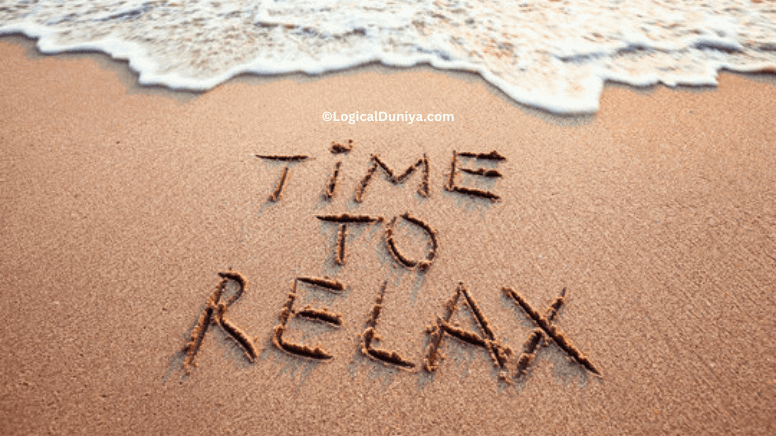
( You have learnt multiple concepts so far⦠Great !!! Take a break for a minute. . )
[Solution – 3] Fluent Wait
Fluent Waits are pretty much similar to Explicit Waits. The Fluent Waits allow you to define polling intervals and exceptions to ignore during the waiting period.
Code Example :
Wait<WebDriver> theWait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
WebElement element = theWait.until(
ExpectedConditions.presenceOfElementLocated(By.id("elementId"))
);
Code Explanation – Step by Step :
The above Selenium code snippet shows the use of Fluent Wait, a more flexible way to implement explicit waits in Selenium. Fluent Wait allows you –
- To specify the maximum amount of time to wait,
- The polling interval, and
- Exceptions that you want to ignore during the wait.
This code example sets up a Fluent Wait with a maximum timeout of 10 seconds, a polling interval of 500 milliseconds, and ignoring NoSuchElementException
. It then waits for an element with the id
attribute “elementId” to be present in the DOM. Once the element is found, it is assigned to the element
variable for further actions.
For more details of this solution on ‘Wait for page load in Selenium – Java‘, do check below points –
1. Wait<WebDriver> theWait = new FluentWait<>(driver) ...
:
Wait<WebDriver>
is an interface representing a waiting strategy in Selenium.FluentWait<>(driver)
creates a new Fluent Wait instance for the specified WebDriver (driver
in this case).
2. .withTimeout(Duration.ofSeconds(10))
:
.withTimeout(...)
sets the maximum amount of time (timeout) to wait for the expected condition to be met. In this case, it’s set to 10 seconds.
3. .pollingEvery(Duration.ofMillis(500))
:
.pollingEvery(...)
specifies the polling interval, which is the frequency at which Selenium checks for the expected condition. Here, it’s set to check every 500 milliseconds (0.5 seconds).
4. .ignoring(NoSuchElementException.class)
:
.ignoring(...)
allows you to specify exceptions that should be ignored during the wait. In this case, it’s configured to ignore theNoSuchElementException
, which commonly occurs when an element is not found.
5. WebElement element = theWait.until(...)
:
theWait.until(...)
waits until the provided expected condition is met. It returns a reference to the web element that meets the condition.ExpectedConditions.presenceOfElementLocated(By.id("elementId"))
is the expected condition. It waits for an element with the givenid
attribute (“elementId”) to be present in the DOM.
Using Fluent Wait provides more control over wait conditions, making your Selenium automation more robust and adaptable to different scenarios. This way, even if your web page takes some time to load, your script will wait for the specified time, will keep checking in every seconds or specified polling time, and will not throw any exception until the time gets over.
[Solution – 4] Page Load Wait
Another solution to let script wait for page load in Selenium – Java, is using the pageLoadTimeout(..)
method. Let’s first see the solution script –
Code Example :
driver.manage().timeouts().pageLoadTimeout(Duration.ofSeconds(10));
Code Explanation – Step by Step :
Selenium code snippet configures the page load timeout for the WebDriver instance. Page load timeout determines the maximum amount of time Selenium should wait for a web page to load completely before it considers the page load as failed.
Here’s an explanation of the code snippet:
1.. driver.manage()
:
driver
is the instance of WebDriver that you’re using for browser automation.manage()
is a method that allows you to configure various settings related to the WebDriver instance.
2. timeout()
:
timeouts()
is a method that allows you to configure different types of timeouts in Selenium, such as page load timeout, script timeout, and implicit wait timeout.
3. pageLoadTimeout(10, TimeUnit.SECONDS)
:
pageLoadTimeout(...)
is used to set the page load timeout.10
is the maximum amount of time you want to wait for a page to load. In this case, it’s set to 10 seconds.Duration.ofSeconds(10)
specifies the time unit for the timeout value, which is seconds in this case.
In summary, this Selenium code sets a page load timeout of 10 seconds for the WebDriver instance (driver
). If a web page doesn’t load completely within this time frame, Selenium will consider it a page load failure. This is a useful configuration to prevent the script from waiting indefinitely for a page to load and to handle scenarios where a page might take too long to load.
Preferred way to handle wait for Page Load
In this well explained tutorial to handle wait for complete page load in Selenium-Java, we learnt more than one ways to handle page load wait time, but NOT all the solutions are preferred to implement in any automation related task. I’ve checked so many other blogs, official Selenium Docs, and I’ve concluded that Explicit Wait and Fluent Wait are the ones which are highly recommended to implement.
Because, the Implicit Wait does not have enough documentations. Also, if you use both – Implicit Wait and Explicit Wait, you will observe uncertain – unwanted behaviours, resulting in extra waiting time.
Similar drawbacks are there with page load timeout solution, So avoid using these two solutions.
Conclusion
Today we have learnt 4 ways to let our script Wait for page load in SeleniumβJava. We now know 4 solutions to handle web page wait – Implicit Wait, Explicit Wait, Fluent Wait and Page Load Wait. At the end, We have also learnt what is most preferred solutions among these available solutions.
Hope this Selenium post on βHow to Wait for Page Load in Selenium – Javaβ has helped to understand it thoroughly. Still, If you have any query related to Selenium, Programming, Resume or Career, just drop your query now β in the below comments section, and Iβll try my best to provide answer to your query within 24 hours.
Also, There is a complete Selenium Framework Mastery Series, in which you will learn a lot of such interesting Selenium Concepts. So, Do check it now.
Keep Thinking Logical . .
Team LogicalDuniya.com
Cheers, Shubham π
This article very help full