Think of a scenario, where you are trying to test email sending automation using Gmail web application. After login, the Gmail’s home page would take some time based on your internet speed and data on Gmail to load completely. So, you need to know – How to get Selenium to Wait for a Page to Load?
In this tutorial, we will learn about this same issue i.e. – How to wait for page loading in Selenium? So, without any delay let’s get started with help of our Selenium Framework Mastery Series –
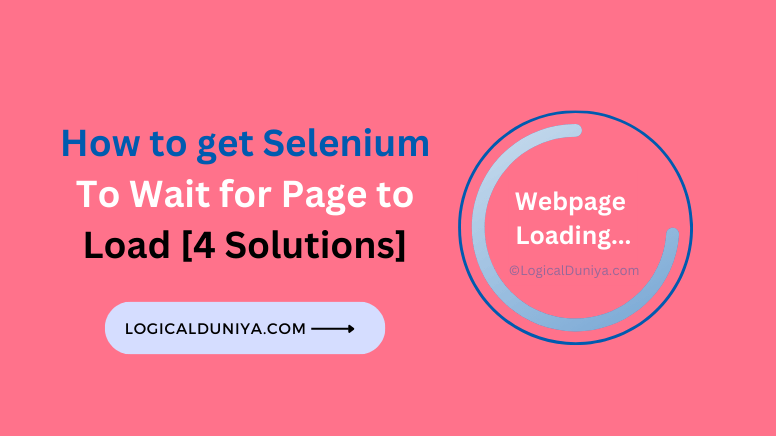
How to get Selenium to Wait for a Page to Load?
There can be multiple solutions of this issues. But, not all of them is advised to implement. We will see further in this tutorial, which of the solutions is highly preferred to be use on – How do I make Selenium wait for some time?
4 Solutions :
- Implicit Wait —> [Less Preferred]
- Explicit Wait (WebDriverWait) —> [Mostly Preferred]
- Fluent Wait —> [Mostly Preferred]
- Page Load Timeout —> [Less Preferred]
The latest version of Selenium provides various useful and simple mechanisms to handle waiting in your test automation scripts. Let’s see in details with example of each of the above solutions:
1. Implicit Wait :
- Purpose:
Implicit waits are used to set a default waiting time for the WebDriver to locate elements. If an element is not immediately found, the WebDriver will wait for the specified time before throwing an exception. The implicit waits are less preferred to implement, because of their uncertain behaviour and less documentations.
- Example:
// Set a Global implicit wait of 20 seconds
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// Find an element; WebDriver will wait up to 20 seconds for it to be located, including if the web page does not get loaded immediately
WebElement myElement = driver.findElement(By.id("theElementId"));
Details :
Above is an example to use the method – implicitlyWait(..)
This is a build-in method of Selenium to implement waiting strategy for Scripts. This is a global wait, which simply means that it applies to every element location call for the entire session.
Its default waiting time is 0
seconds, which means that if the element is not found at runtime, it will immediately return / throw a Selenium Error.
2. Explicit Wait (WebDriverWait):
- Purpose:
Explicit waits are used when you want to wait for a specific condition to be met before proceeding with further actions. You can specify the maximum wait time and the expected condition to wait for. Remember, If the condition is not met before the given timeout value, the code will give a timeout error.
Explicit waits (such asWebDriverWait
) are a great choice to specify the exact condition to wait for in each place it is needed. Another nice feature is that, by default, the Selenium Wait class automatically waits for the designated element to exist. That’s why, Explicit waits are preferred over implicit wait, when if need to handle slow web page loading. - Example:
// Step 1 - Create a WebDriverWait instance with a timeout of 20 seconds
WebDriverWait theWait = new WebDriverWait(driver, 20);
// Step 2 - Wait for an element with the specified condition
WebElement element = theWait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Details :
The above Selenium code snippet is using explicit waiting to wait for the visibility of an element with the specified id
. Let’s understand the example step by step:
Step 1 – Create Wait :
- In above example, We have used
WebDriverWait theWait = new WebDriverWait(driver, 20);
to create an explicit wait with 20 seconds timeout. It is tied with the current session with help of givendriver
instance.
Step 2.a – WebElement element
:
- In above example, This line declares a variable named
element
of typeWebElement
. This variable will store the reference to the web element once it becomes visible on the web page.
Step 2.b – theWait.until(...)
- In above example,
theWait
is a reference to aWait<WebDriver>
instance, which is typically created using explicit wait techniques likeWebDriverWait
. .until(...)
is a method used with explicit waits. It waits for a specific condition to be met.
Step 2.c – ExpectedConditions.visibilityOfElementLocated(By.id("elementId"))
:
- In above example, we have used
ExpectedConditions
. It is a class in Selenium that provides a collection of expected conditions that can be used with explicit waits. visibilityOfElementLocated(...)
is an expected condition that checks for the visibility of an element located by a given selector. In this case, it’s locating an element by itsid
attribute (“elementId”).
// Step 1 - Create a WebDriverWait instance with a timeout of 20 seconds
WebDriverWait theWait = new WebDriverWait(driver, 20);
// Step 2 - Wait for an element with the specified condition
WebElement element = theWait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
So overall, if the web page gets loaded within 20 seconds, what the code does is as follows –
- It uses explicit waiting to wait for an element with the
id
attribute “elementId” to become visible on the web page. - Once the element is found and is visible, it returns a reference to that element and assigns it to the
element
variable for further operations to be performed.
This approach ensures that the script waits until the desired element is both present in the DOM and visible on the web page before attempting to interact with it. It helps in handling synchronization issues where elements may not be immediately available when the page loads.
3. Fluent Wait:
- Purpose
Fluent waits are an extension of explicit waits that provide more flexibility. They allow you to define both the maximum wait time and the polling interval (how frequently Selenium checks the condition). You can also configure exceptions to ignore during the wait.
- Example:
// Step 1- Create a FluentWait instance with a timeout of 20 seconds and a polling interval of 500 milliseconds
FluentWait<WebDriver> theFluentWait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(20))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
// Step 2- Wait for an element with the specified condition
WebElement element = theFluentWait.until(ExpectedConditions.presenceOfElementLocated(By.id("ourElementId")));
Details :
In the above Selenium code, we are using FluentWait, which is a super flexible way to wait for an element with a specific condition. With help of it, we will know – How to get Selenium to Wait for a Page to Load? Let’s understand it’s implementation steps in simple terms:
Step 1 – Create a FluentWait Instance:
In this step, we’re setting up how long we’re willing to wait for an element to meet a certain condition.
FluentWait<WebDriver> theFluentWait = new FluentWait<>(driver)
:
We create a FluentWait instance and associate it with our WebDriver instance (driver
). This tells Selenium which browser to wait for..withTimeout(Duration.ofSeconds(20))
:
We set a maximum waiting time of 20 seconds. This means we’re willing to wait up to 20 seconds for our condition to be met..pollingEvery(Duration.ofMillis(500))
:
We specify how often Selenium checks if the condition is met. It checks every 500 milliseconds (half a second). This means it won’t continuously check but rather at regular intervals..ignoring(NoSuchElementException.class)
:
We specify which exceptions to ignore during the waiting period. In this case, we’re telling Selenium to ignore the “NoSuchElementException,” which can occur when an element is not found.
Step 2 – Wait for the Condition:
Here, we’re specifying the condition we want to wait for.
WebElement element = theFluentWait.until(ExpectedConditions.presenceOfElementLocated(By.id("ourElementId")))
: We’re telling Selenium to wait until an element with theid
“ourElementId” is present on the page. Once it’s found, it assigns a reference to this element to theelement
variable.
So, overall, the above Fluent Wait code example sets up a wait with a maximum timeout of 20 seconds. It checks every 500 milliseconds for an element with a specific ID to appear on the page. If it finds the element within the timeout, it stores a reference to that element in the element
variable. If it doesn’t find it within 20 seconds, it will throw a timeout exception.
This is useful for handling dynamic page content or ensuring that an element loads before interacting with it.
4. Page Load Timeout:
- Purpose: Page load timeout is used to specify the maximum amount of time Selenium should wait for a web page to load completely. It ensures that your script doesn’t wait indefinitely for a page to load.
- Example:
// Set a page load timeout of 20 seconds
driver.manage().timeouts().pageLoadTimeout(20, TimeUnit.SECONDS);
// Navigate to a web page; WebDriver will wait up to 20 seconds for the page to load
driver.get("https://your-example.com");
Details :
The pageLoadTimeout(..)
method sets the time interval in which web page needs to be loaded in a current browsing context. When a new session is created by WebDriver, the default timeout 300,000 gets imposed. If page load limits a given/default time frame, the script will be stopped by throwing – TimeoutException.
Conclusion
In this in-depth and step by step tutorial, we have learnt about – How to get Selenium to Wait for a Page to Load? We have learnt about 4 solutions and each of the page load waiting strategy and waiting mechanisms serves a specific purpose and can be used based on your automation requirements. They help make your tests more reliable and robust by handling synchronization issues and waiting for elements or conditions to be ready before performing actions even if the web page is not loading on time.
Hope, now you have some ideas / solutions on How do I make Selenium wait for some time? or Wait for page load in Selenium – Java. Still, If you have any query related to Selenium, Programming, Resume or Career, just drop your query now – in the below comments section, and I’ll try my best to provide answer to your query within 24 hours.
Also, There is a complete Selenium Framework Mastery Series, in which you will learn a lot of such interesting Selenium Concepts. So, Do check it now.
Keep Thinking Logical . .
Team LogicalDuniya.com
Cheers, Shubham 🙂