If you looking for a simple and step-by-step Selenium Tutorial to explain – How to check if element is present, in Selenium-Java? Then you have come on the right place. In this detailed Selenium tutorial, we will learn about 4 different ways to write test script solutions on – How to check if element exists with Java-Selenium?
So, Let’s continue with our Selenium Framework Mastery Series –
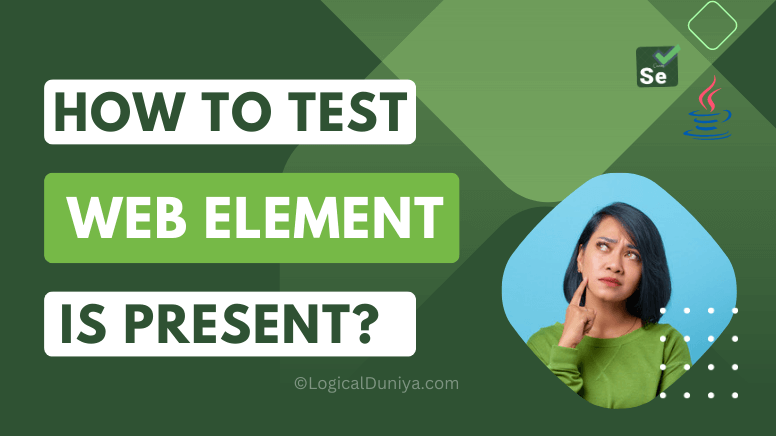
Test if an Element is Present using Selenium
In Selenium WebDriver, there are multiple ways to find out if an element is present on web page or not. Those solutions can be implemented using –
findElement()
– Selenium WebDriver method.findElements()
– Selenium WebDriver method.WebDriverWait
– Selenium WebDriver interface.JavascriptExecutor
– Selenium WebDriver interface.
Let’s see simple examples and step by step explanations for each of the above solutions on – How to check if element is present in Selenium-Java?
Test script with 4 Solutions – How to find Element in Selenium + Java?
package com.logicalduniya.practice.selenium;
// Step 1: Import necessary Classes and Interfaces -
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.NoSuchElementException;
// Step 2 - Created a Test Class -
public class CheckElementPresence {
// Step 3 - Declaring the WebDriver reference variable -
static WebDriver driver;
// Step 4 - The main() method -
public static void main(String[] args) throws Exception {
// Step 5 - Setting up the Chrome Browser Driver instance
// To execute this code in your system, You need to change the below second parameter as per your chromedriver file location..
System.setProperty("webdriver.chrome.driver",
"/Users/logicalduniya_com/Documents/Plugins/chromedriver");
ChromeOptions opts = new ChromeOptions();
opts.addArguments("--remote-allow-origins=*");
// Created the ChromeDriver object with configured chrome options -
driver = new ChromeDriver(opts);
// Step 6 - Open the web page
driver.get("https://www.google.com");
// Waiting for 5 seconds to let the web page load properly..
Thread.sleep(5000);
// Step 7 - Created 4 methods to Verify whether the element is present or not -
verifyElementPresentWay1();
verifyElementPresentWay2();
verifyElementPresentWay3();
verifyElementPresentWay4();
// Step 8 - Close the browser driver object -
driver.quit();
}
// Solution 1 -
private static void verifyElementPresentWay1() {
// Locate the Google Search button -
WebElement element = driver.findElement(By.name("btnK"));
System.out.println("\n\n---- Solution 1 : findElement() : ");
// Check if the element is present -
if (element != null) {
System.out.println("Element is PRESENT on the page");
} else {
System.out.println("Element is NOT PRESENT on the page");
}
}
// Solution 2 -
private static void verifyElementPresentWay2() {
// Locate the Google Search button -
List<WebElement> element = driver.findElements(By.name("btnK"));
System.out.println("\n\n---- Solution 2 : findElements() : ");
// Check if the element is present -
if (element != null & element.size() > 0 ) {
System.out.println("Element is PRESENT on the page");
} else {
System.out.println("Element is NOT PRESENT on the page");
}
}
// Solution 3 -
private static void verifyElementPresentWay3() {
// Creating the WebDriverWait Object with 5 seconds timeout duration -
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(5));
wait.ignoring(NoSuchElementException.class);
// An expectation for checking that the given element is present on the DOM of a page -
wait.until( ExpectedConditions.presenceOfElementLocated(By.name("btnK")) );
// Getting the desired WebElement -
WebElement element = driver.findElement(By.name("btnK"));
System.out.println("\n\n---- Solution 3 : WebDriverWait : ");
if (element != null) {
System.out.println("Element is PRESENT on the page");
} else {
System.out.println("Element is NOT PRESENT on the page");
}
}
// Solution 4 -
private static void verifyElementPresentWay4() throws Exception {
// Preparing the Javascript code to get the WebElement -
String javascript = "document.querySelector('input[name=\"btnK\"]');";
// Created the JavascriptExecutor instance -
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
// Executing the prepared JavaScript code using JavascriptExecutor -
WebElement element = (WebElement) jsExecutor.executeScript(javascript);
System.out.println("\n\n---- Solution 4 : JavascriptExecutor : ");
if (element != null ) {
System.out.println("Element is PRESENT on the page");
} else {
System.out.println("Element is NOT PRESENT on the page");
}
}
}
Output
---- Solution 1 : findElement() :
Element is PRESENT on the page
---- Solution 2 : findElements() :
Element is PRESENT on the page
---- Solution 3 : WebDriverWait :
Element is PRESENT on the page
---- Solution 4 : JavascriptExecutor :
Element is PRESENT on the page
POM.xml file –
If you are using maven based Java project, then below pom.xml file will be required. It contains a few of the required dependencies (plugins), from which we have taken some java classes in above test script solution.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.logicalduniya</groupId>
<artifactId>LogicalDuniyaSoftwareTesting</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.4.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/io.github.bonigarcia/webdrivermanager -->
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>5.3.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Step by Step – Test Code Explanation
The above test script gives us 4 solutions on – How do you check if an element is present in Selenium? So, Let’s break down the above script and explain each important section of it step-by-step :
A] Import statements:
package com.logicalduniya.practice.selenium;
// Step 1: Import necessary Classes and Interfaces -
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.NoSuchElementException;
The script starts with importing necessary packages and classes from the Selenium library to facilitate web testing.
Here we have imported predefined java classes from Java and few classes from Selenium framework. We have imported 2 classes – java.time.Duration
and java.util.List
from Java library, while all the other import statement are to fetch some Selenium library classes.
B] WebDriver Setup:
// Step 2 - Created a Test Class -
public class CheckElementPresence {
// Step 3 - Declaring the WebDriver reference variable -
static WebDriver driver;
// Step 4 - The main() method -
public static void main(String[] args) throws Exception {
// Step 5 - Setting up the Chrome Browser Driver instance
// To execute this code in your system, You need to change the below second parameter as per your chromedriver file location..
System.setProperty("webdriver.chrome.driver",
"/Users/logicalduniya_com/Documents/Plugins/chromedriver");
ChromeOptions opts = new ChromeOptions();
opts.addArguments("--remote-allow-origins=*");
// Created the ChromeDriver object with configured chrome options -
driver = new ChromeDriver(opts);
...
...
...
}
}
In above test script, from Step 2 to Step 5 –
- We created a test class :
CheckElementPresence
- Then, we declared a static variable
driver
of typeWebDriver
. This variable isstatic
in nature, so it will be available among all the static blocks and static methods of the current test class. - Then, in
main()
method, we set up theWebDriver
for Chrome browser, by setting the system property"webdriver.chrome.driver"
with the path to the downloadedChromeDriver
binary file. - We then create a new instance of the
ChromeDriver
with requiredChromeOptions
. - Then, the statement
opts.addArguments("--remote-allow-origins=*");
with ChromeDriver allows the Chrome browser to make cross-origin requests during test automation. - Cross-origin requests are requests made by a web page to a different domain or origin than the one from which the page itself originated. Browsers usually restrict cross-origin requests due to security concerns, as it can potentially lead to data leakage or unauthorized access to resources.
- Then, we instantiated the
ChromeDriver()
object by passing the configuredChromeOptions
with variableopts
.
C] Open the Web Page:
At step 6, you can see we are launching the google.com webpage using the get()
method of WebDriver
. The script opens a Google homepage by navigating to “https://www.google.com”.
D] Hard Wait for Page Load:
Then, we added a 5-second pause, using Thread.sleep()
. It stops the execution and asks the WebDriver object to wait for 5 seconds before performing any other statements.
E] Verification Methods:
Then in the script, we defined four different custom created methods to check if element is present – method 1 = verifyElementPresentWay1()
, method 2 = verifyElementPresentWay2()
, method 3 = verifyElementPresentWay3()
, method 4 = verifyElementPresentWay4()
to check if the element is present on the page using different approaches.
E.1] verifyElementPresentWay1()
: This method uses the findElement()
method to locate the Google Search button by its name
attribute and checks if the element is not null to determine its presence.
E.2] verifyElementPresentWay2()
: This method uses the findElements()
method to locate the Google Search button by its name
attribute. It retrieves a list of matching elements and checks if the list is not null and has a size greater than 0 to determine the element’s presence.
E.3] verifyElementPresentWay3()
: This method uses WebDriverWait
along with the ExpectedConditions.presenceOfElementLocated()
method to wait for the presence of the Google Search button. It then uses the findElement()
method to locate the element and checks if it is not null to determine its presence. We will learn more about WebDriverWait
in our Selenium Framework Mastery Series.
E.4] verifyElementPresentWay4()
: This method utilizes JavaScript execution through the JavascriptExecutor
interface. It executes a JavaScript code snippet to locate the Google Search button using a CSS selector and checks if the element is not null to determine its presence.
F] Output and Closure:
After checking element presence using above 4 different methods, the script prints the results. Finally, it closes the browser window using driver.quit()
to end the WebDriver
session.
Conclusion
In the above test script demonstrates various techniques to verify element presence, including using findElement()
, findElements(), WebDriverWait
, and JavaScript execution. Each method provides a different mechanism to validate the presence of an element on the web page.
The above Selenium script can further be modified to work more efficiently, but to keep it simple for Selenium beginner, I’ve kept it like this only. Hope you have understood now – How to check if element is present? In Selenium-Java? If you have any question / suggestion, then please mention them in comment seciton below, I’ll try to answer your query, within 24 hours.
Cheers,
Shubham.