JavaScript Variable Hoisting is a fundamental concept that every JavaScript developer must understand. This unique behavior of JavaScript can lead to unexpected results if not properly comprehended.
In this guide of JavaScript Mastery Series, we will break down JavaScript variable hoisting step by step, using examples and explanations to make it easier for you to understand. Whether you’re just starting or preparing for an interview, this guide covers everything you need to know. So, Let’s get started –
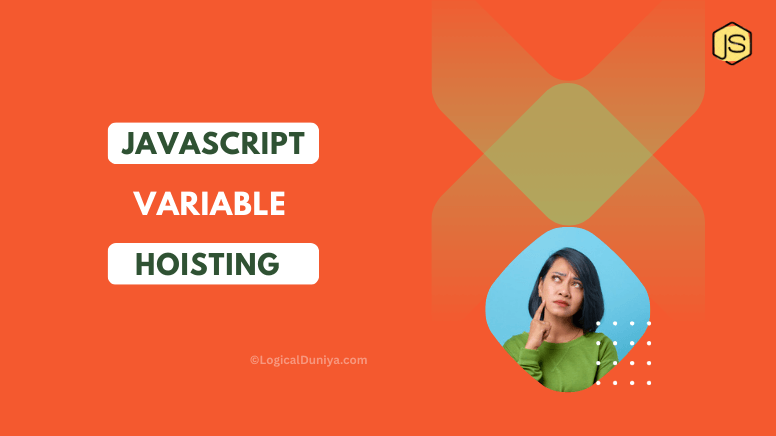
JavaScript Hoisting Explained
In our projects, we most of the time write (or declare) functions, that we call and execute later. Hoisting in JavaScript is the default behavior of moving declarations to the top of their scope. If you have written body of a JavaScript function in the middle, then JavaScript Engine will cut from there and put it at the top.
When JavaScript executes code, it “hoists” variable, function, and class declarations to the top of their respective scopes before the code runs.
console.log(a); // Output: undefined
var a = 5;
In the above example, JavaScript interprets the code as:
var a; // Variable hoisted at top by JS Engine
console.log(a); // Output: undefined
a = 5;
This happens because the declaration var a
is hoisted to the top, while the assignment remains in place.
JavaScript Hoisting Variables
Variables in JavaScript are hoisted differently depending on how they are declared (var
, let
, or const
).
var
: Declarations are hoisted and initialized with a value calledundefined
.let
andconst
: Declarations are hoisted but are not initialized. Accessing them before initialization results in an error, which isReferenceError
.
console.log(myMsg); // Output => undefined
var myMsg = 'My LogicalDuniya';
console.log(yourMsg); // Output => ReferenceError: Cannot access 'yourMsg' before initialization
let yourMsg = 'Your LogicalDuniya';
Interview Tipπ‘:
In interviews, you might be asked howlet
andconst
differ in hoisting behavior compared tovar
. So, prepare this concept pretty well.
JavaScript Hoisting Definitions
To clearly define hoisting:
- What is hoisted?
Variable and function declarations are hoisted. - What is NOT hoisted?
Variable assignments and function expressions are not hoisted.
Example:
console.log(myFunc()); // Output: "Hello!"
function myFunc() {
return "Hello LogicalDuniya.com!";
}
console.log(yourFunc()); // Output: TypeError: yourFunc is not a function
var yourFunc = function () {
return "Hii LogicalDuniya.com!";
};
JavaScript Hoisting and Scope
Hoisting behaves differently in global scope and function scope.
Example of hoisting in global scope:
console.log(a); // undefined
var a = 10;
Example of hoisting in function scope:
function example() {
console.log(b); // undefined
var b = 20;
}
example();
Types of Hoisting in JavaScript
Variable Hoisting: Variables declared with var
are hoisted and initialized with undefined
.
Function Hoisting: Function declarations are fully hoisted and can be called before their definition.
Class Hoisting: Classes are hoisted but are not initialized.
Here’s a simple example of class hoisting in JavaScript:
// This will throw an error
const myDog = new Animal("Buddy");
class Animal {
constructor(name) {
this.name = name;
}
}
Key Points About Class Hoisting in JavaScript :
- Classes are hoisted, but they behave differently from function declarations.
- Unlike function hoisting, you cannot use a class before its declaration.
- Attempting to instantiate a class before its declaration results in a
ReferenceError
. - The error message will be: “Cannot access ‘Animal’ before initialization”.
Let me also tell you the Correct Way to Use the Class in JavaScript :
class Animal {
constructor(name) {
this.name = name;
}
}
// Now you can create an instance
const myDog = new Animal("Buddy");
Interview Tipπ‘:
In interviews, they ask this question -How will you avoid hoisting-related issues?
Then, you need to answer :- To avoid hoisting related issue, we should always declare our classes before using them. This ensures predictable and error-free code execution.
The key difference is that while function declarations are fully hoisted and can be called before their actual declaration, classes exist in a “temporal dead zone” similar to let
and const
variables, meaning they must be declared before they can be used.
JavaScript Hoisting Var, Let, Const
Let’s understand it clearly –
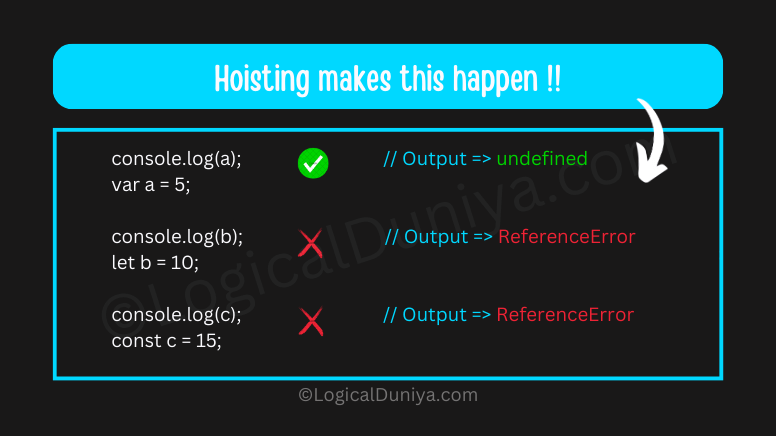
var
: Hoisted and initialized withundefined
.let
: Hoisted but not initialized.const
: Hoisted but must be initialized during declaration.
Example :
console.log(a); // Output => undefined
var a = 5;
console.log(b); // Output => ReferenceError
let b = 10;
console.log(c); // Output => ReferenceError
const c = 15;
JavaScript Hoisting Exercises for Beginners
Let me give you some exercises to test your understanding:
1) What will the following code output?
console.log(a);
var a = 10;
console.log(b);
let b = 20;
Answer: undefined
ReferenceError
.
2) Predict the output:
LogicalDuniyaFunc();
function LogicalDuniyaFunc() {
console.log("Hoisted!");
}
Answer: "Hoisted!"
3) True or False: let
and const
declarations are initialized as undefined
during hoisting.
Answer:
False. They are hoisted but not initialized.
Interview-Level Tips
- Understand Temporal Dead Zone (TDZ):
Forlet
andconst
, the TDZ refers to the time between entering the scope and the variable being initialized. - Function Expressions vs. Declarations:
In interviews, differentiate between the hoisting of function declarations and function expressions. - Avoid
var
in Modern JavaScript:
Explain whylet
andconst
are preferred due to their predictable scoping and hoisting behavior.
What we had learnt today?
In this simple guide, we explored an interesting concept of JavaScript Variable Hoisting, understanding how JavaScript moves variable and function declarations to the top of their scope during the compilation phase.
We understood the behavior of hoisting in var, let, and const, highlighting key differences and the impact of the Temporal Dead Zone (TDZ). We had also discussed topics like hoisting and scope, types of hoisting in JavaScript with practical examples and interview tips for all JavaScript Developers.
- To learn more simple and detailed JavaScript guides, do check our JavaScript Mastery Series.
- To use hundreds of free tools to fasten your daily development and testing tasks, visit β Tools.LogicalDuniya.com
- To know about more tutorials of other latest technologies & concepts, visit our Tutorials page.
Happy Learning, Cheers..
Shubham : )