Operators in JavaScript are symbols or keywords that instruct the interpreter to perform specific actions on values and variables. These actions can include arithmetic calculations, assignments, comparisons, or logical operations.
In this guide of JavaScript Mastery Series, we will learn about a few important operators in JavaScript, including JavaScript Arithmetic Operators, JavaScript Assignment Operators, JavaScript Comma Operator, JavaScript Unary Operators and others. So, Let’s get started –
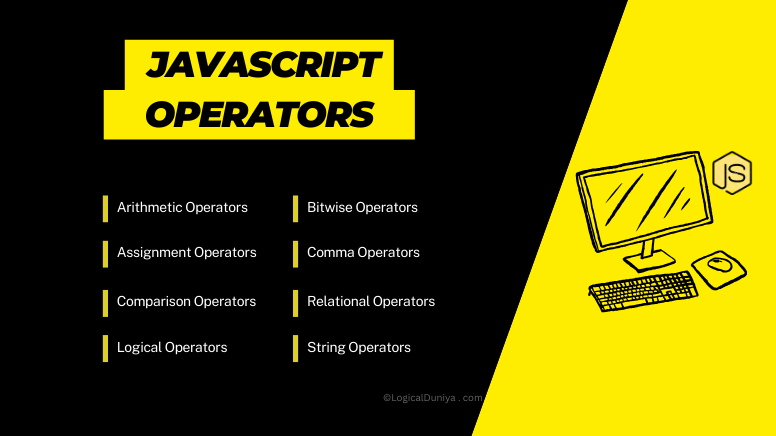
JavaScript Operators: Types, Examples & Use Cases
Operators in JavaScript are symbols or keywords that instruct the interpreter to perform specific actions on values and variables. These actions can include arithmetic calculations, assignments, comparisons, or logical operations.
Example :
let a = 10;
let b = 15;
console.log(a + b); // Outputs: 25
Operator Precedence in JavaScript
JavaScript does not execute any operator on any order. It uses a rule that is called Operator Precedence. Operator precedence determines the order in which operations are evaluated.
For example, multiplication and division are performed before addition and subtraction. Parentheses can be used to override precedence.
Example:
let result = 10 + 25 * 2;
console.log(result); // Outputs: 60 (multiplication first)
result = (10 + 25) * 2;
console.log(result); // Outputs: 70 (parentheses first)
Interview Tipπ‘:
In interviews, Understanding operator precedence helps avoid bugs in complex expressions given by your interviewer.
JavaScript Arithmetic Operators
Arithmetic operators are used to perform basic math operations. Here’s a summary table –
Operator | Description | Example | Output |
+ | Addition | 5+3 | 8 |
- | Subtraction | 5-3 | 2 |
* | Multiplication | 5*3 | 15 |
/ | Division | 5/2 | 2.5 |
% | Modulus | 5%2 | 1 |
Example :
let x = 10;
let y = 3;
console.log(x % y); // Outputs: 1
JavaScript Assignment Operators
Assignment operators are used to assign values to variables.
Operator | Example | Equivalent |
= | x = 5 | x = 5 |
+= | x += 5 | x = x + 5 |
-+ | x -= 5 | x = x – 5 |
*= | x *= 5 | x = x * 5 |
Example :
let x = 10;
x += 5; // x = 15
console.log(x); // Outputs: 15
JavaScript Comparison Operators
Comparison operators compare two values and return a Boolean result.
Operator | Description | Example | Output |
== | Equal To | 5 == ‘5’ | true |
=== | Strict Equal To | 5 === ‘5’ | false |
!= | Not Equal To | 5 != ‘5’ | false |
!== | Strict Not Equal To | 5 !== ‘5’ | true |
Example:
console.log(10 === '10'); // Outputs: false
console.log(10 == '10'); // Outputs: true
JavaScript Logical Operators
Logical operators are used to perform logical operations and return Boolean values.
Operator | Description | Example | Output |
&& | Logical AND | true or false | |
|| | Logical OR | <code>true or false | |
! | Logical NOT | <code>true or false |
Example:
// JavaScript Logical Operators
let a = true;
let b = false;
console.log(a && b); // Logical AND: false (both need to be true for true)
console.log(a || b); // Logical OR: true (only one needs to be true)
console.log(!a); // Logical NOT: false (negates the value of 'a')
JavaScript Bitwise Operators
Bitwise operators work at the binary level, manipulating individual bits.
// JavaScript Bitwise Operators
let x = 5; // Binary: 0101
let y = 3; // Binary: 0011
console.log(x & y); // Bitwise AND: 1 (0101 & 0011 -> 0001)
console.log(x | y); // Bitwise OR: 7 (0101 | 0011 -> 0111)
console.log(x ^ y); // Bitwise XOR: 6 (0101 ^ 0011 -> 0110)
console.log(~x); // Bitwise NOT: -6 (~0101 -> 1010 in two's complement)
console.log(x << 1); // Left Shift: 10 (0101 << 1 -> 1010)
console.log(x >> 1); // Right Shift: 2 (0101 >> 1 -> 0010)
JavaScript Ternary Operators
The ternary operator is a shorthand for if-else
.
Syntax :
condition ? valueIfTrue : valueIfFalse;
Example :
let age = 18;
let canVote = age >= 18 ? "Yes" : "No";
console.log(canVote); // Outputs: Yes
JavaScript Unary Operators
Unary operators operate on a single operand.
Example :
let x = 5;
console.log(-x); // Outputs: -5
console.log(typeof x); // Outputs: number
JavaScript in
and instanceof
Operators
The in
operator checks if a property exists in an object. The instanceof
operator checks if an object is an instance of a specific class.
let obj = { name: "John" };
console.log("name" in obj); // Outputs: true
let arr = [];
console.log(arr instanceof Array); // Outputs: true
JavaScript String Operators
String operators include concatenation (+
) and concatenation assignment (+=
).
Example :
let greeting = "Hello";
let name = "World";
console.log(greeting + " " + name); // Outputs: Hello World
What Did We Learn Today ?
In this comprehensive guide, we explored the various types of JavaScript Operators essential for mastering JavaScript. Starting with operator precedence, we saw how different operators follow specific rules for evaluation. Also we have written program on various operators such as JavaScript Assignment Operators, JavaScript Comparison Operators, JavaScript Logical Operators, JavaScript Unary Operators and more.
We have also understood a couple of key points to remember in any JavaScript Interview. I would recommend to keep experimenting with these operators to enhance your skills and boost your expertise in JavaScript development. Next –
- To learn more simple and detailed JavaScript guides, do check our JavaScript Mastery Series.
- To use hundreds of free tools to fasten your daily development and testing tasks, visit β Tools.LogicalDuniya.com
- To know about more JS Tutorials and other latest technologies & concepts, visit our Tutorials page.
Happy Learning, Cheers!!!
Shubham : )
Great guide