In this detailed guide, you will understand about an important Java Interview Question : Java pass-by-reference. Today, we will learn – Can Java perform pass by reference? and, Is Java pass by value or pass-by-reference? So, Let’s get started and dive deeper into it.
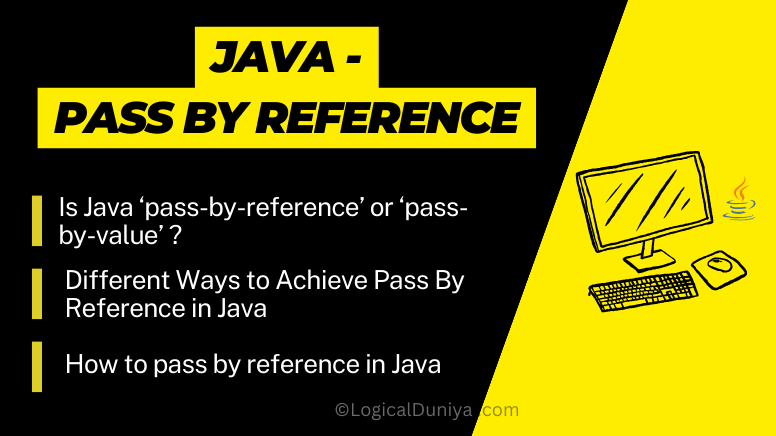
Java pass-by-reference
Let me first clear the doubt – Java only supports pass-by-value. Always. 100%. Java only supports pass-by-value and DOES NOT support pass-by-reference.
Also, in Java – we can pass references as a value. This is what actually creates confusion. Let’s clarify this doubt with an example –
Example 1 – How does Java perform pass-by-value ?
package logical.duniya.com;
// Dummy House Class -
class House {
private String colour;
public String getColour() {
return colour;
}
public void setColour(String newColour) {
colour = newColour;
}
}
// Main Class -
class Main {
public static void main(String[] args) {
System.out.println("-------- Java Pass by Reference & Pass by Value Explanation : by LogicalDuniya.com ---------");
// Created 'House' object and saved its reference in 'myHouse' reference variable -
House myHouse = new House();
myHouse.setColour("Blue");
// Before update -
System.out.println("Before = " + myHouse.getColour());
// Passing reference of House object kept in myHouse reference variable -
changeColor( myHouse );
// After update -
System.out.println("After = " + myHouse.getColour());
}
private static void changeColor(House theHouse) {
theHouse.setColour("Red");
}
}
Output
-------- Java Pass by Reference & Pass by Value Explanation : by LogicalDuniya.com ---------
Before = Blue
After = Red
Explanation – Step by Step
Firstly, we have created a dummy House
class. This class is having a member variable colour
with 2 member methods to set & get the colour
value of House
object –
getColour()
setColour(String newColour)
We said, Java is Pass-By-Value. That’s why, it has printed Before = Blue
// Passing 'reference' of previously created House object stored in myHouse reference variable -
changeColor( myHouse );
// After update -
System.out.println("After = " + myHouse.getColour());
Then, as you can see above, we called changeColor(..)
method and passed the myHouse
object reference (the memory address) as a value in this method changeColor(..)
.
class Main {
public static void main(String[] args) {
...
...
// Passing reference of House object kept in myHouse reference variable -
changeColor( myHouse );
...
...
}
private static void changeColor(House theHouse) {
theHouse.setColour("Red");
}
}
After that, as you can see above, the object reference value has been received by theHouse
reference variable of changeColor(..)
method.
Now this part is important to understand. Both the reference variable myHouse
and theHouse
are now targeting same object, as they are containing the same reference value.
Then, as the changeColor(..)
method performs it’s task and sets the new colour “Red
” targeting the same object whose reference (memory address) has been given to it.
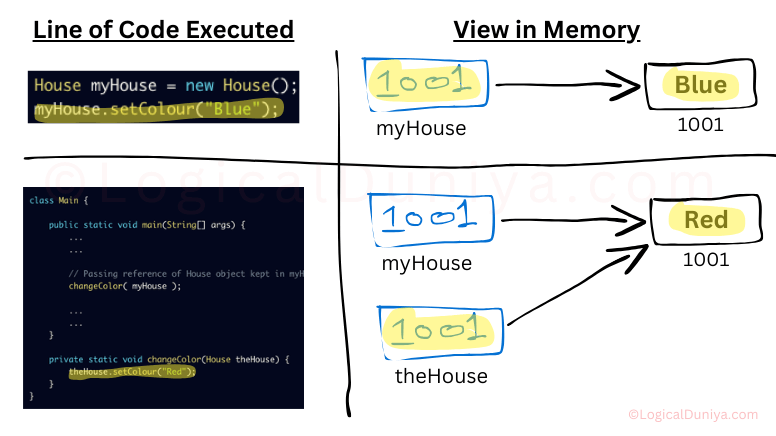
As you can see in above illustration, the changeColor(myHouse)
acted as passing the House object memory address value to the changeColor(..)
method and inside that method, it then updated the colour to “Red
“. So, overall Java supports pass by value and DOES NOT supports pass-by-reference.
If you want to know more about this, just check this FAQ on Oracle Forum For Java. It has said there –
Everything in Java is an Object, and all the objects are passed by reference. BUT, references themselves are passed by value – and not by another reference as it happens.
Conclusion
In this step-by-step tutorial on – Java Pass-By-Reference behind the scene. We have learnt that, the value of reference variable gets send to the called method. Hope you have understood this concept.
If you have any query or suggestions, feel free to comment below, and I will try my best to answer your doubts within 24 hours. You will learn so many concepts of Java, Selenium and various other technologies as well as latest Interview Questions and Answers, So I’ll recommend to Bookmark the LogicalDuniya.com in your favourite browser and visit the Categories section of it.