Frames are web elements which are used to contain multiple other web elements under it. Due to which, even if your web element locator (XPath / CSS Selector etc.) is correct, your Selenium Script will fail to find that web element. That’s why, In this Selenium Framework tutorial, we will learn about – What are Frames in Selenium? and How to handle Frames in Selenium to execute our test script successfully?
This tutorial of Handling iframe
and frame
Web Element, is part of the Selenium Framework Mastery Series. So, in case if you are interested to learn more about other important concepts of Selenium Framework with Illustrations and Simple examples, you should definitely check that series after completing this Frame tutorial post.
So, Let’s get starter with Frames in Selenium –
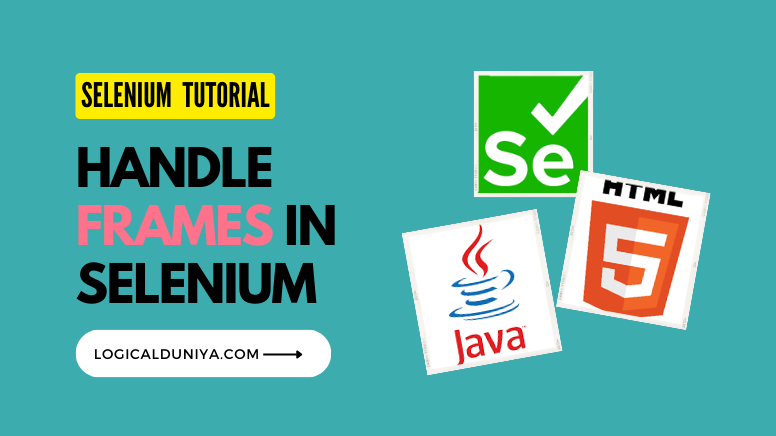
Core Steps : To Handle Frames in Selenium
There is so much things to know around handling frame
/ iframe
web elements. But, most of the times these 2 Selenium statements will help you to jump onto the frame and pick any web element present inside that frame
or iframe
–
driver.switchTo().defaultContent();
driver.switchTo().frame(...); // Here, in place of (...) you can put either Index (int), or frame ID (String) or frame Name (String)
Each frame
or iframe
is like a separate HTML document embedded within the main page. So, to interact with elements inside a frame
or iframe
, you need to switch the WebDriver’s focus to that particular frame
or iframe
. The driver.switchTo().frame(..)
method is used for this purpose.
However, there’s a special case where you might want to switch the WebDriver’s focus back to the main content of the page from within a frame. This is where the driver.switchTo().defaultContent()
method comes into play.
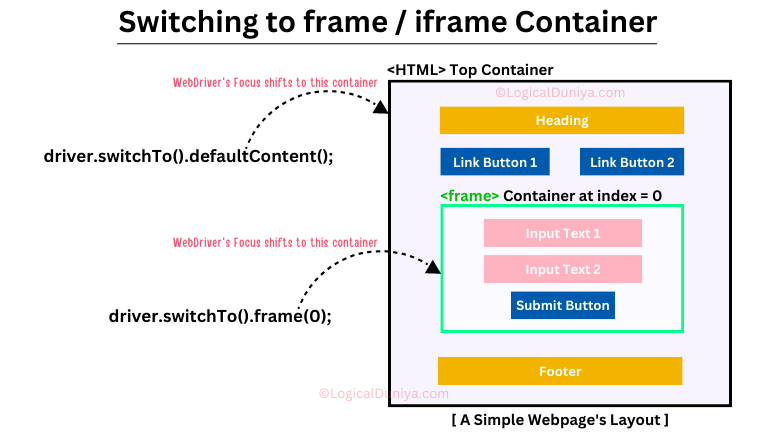
[A] Let’s understand the code statement driver.switchTo().defaultContent()
–
driver
: This is the object of WebDriver you are using to control the browser.switchTo()
: This is a method provided by theWebDriver
to switch its focus to different contexts, such as frames, windows, or the main content.defaultContent()
: This method switches the focus back to the main content of the page (outside anyframe
/iframe
). It essentially tells WebDriver to move out of any frames it might currently be within and focus on the main page.
When you use driver.switchTo().defaultContent()
, WebDriver will no longer be interacting with elements within a frame
/ iframe
. It’s a way to ensure that subsequent interactions with elements are occurring in the main content or top container of the web page.
[B] Let’s understand the code statement driver.switchTo().frame(..)
–
Above we have discussed about driver
object and it’s switchTo()
method. Let’s talk a bit about the frame(..)
method.
Types of frame(..) methods in Selenium
frame(..)
method is the main method used to switch to different frame / iframe
Web Elements. The frame(..)
method in Selenium WebDriver is used to switch the focus of the driver to a specific frame / iframe
(inline frame) within a web page. It allows you to interact with elements inside the selected frame.
The frame(..)
method has several variants based on how you specify the frame to switch to. Here are the variants of the frame(..)
method along with examples: Here is a simple example with HTML Source code and Selenium script –
Source Code :
<html>
<header>
...
</header>
<frame class='frame-login'>
<input placeholder='Username' />
<button>Submit Username</button>
</frame>
<frame class='frame-pwd'>
<button>Forget Password</button>
</frame>
<footer>
...
</footer>
</html>
Observe the above source code. You can assume as – It has a top container with 2 sub-containers ‘ of ‘frame’ web elements. Here is how you need to access web elements inside those Frames in Selenium –
1] Switch to frame by Index :
Let’s say – you have checked the source code of webpage and found that – on the web page there are in total 2 frame
web elements are present as a sibling (brother – sister), then to access web elements inside those frames, you can use indices of those frame
web elements assigned by the DOM.
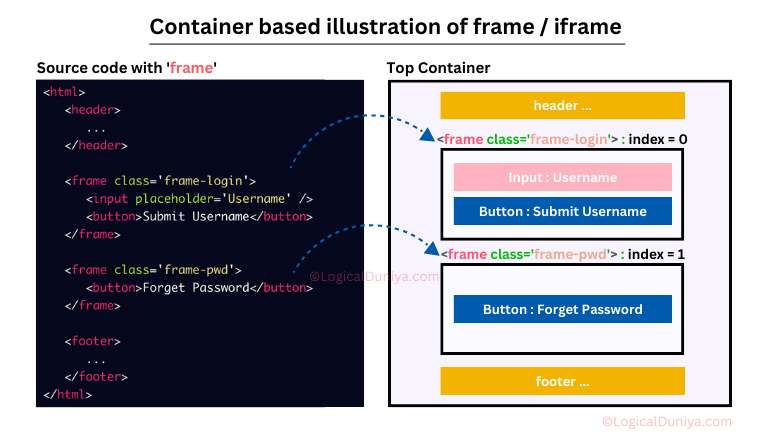
Selenium code : To switch to 1st frame
This 1st frame would be internally having : Index = 0, so the Selenium code to work with elements present inside the frame would be something like :
// Switch to default top container -
driver.switchTo().defaultContent();
// Provide the index = 0
driver.switchTo().frame(0);
// Then perform operation on Web Elements present inside the current frame-
driver.findElement(By.xpath("//input[@placeholder='Username']"))
.sendKeys("LogicalDuniya_User1");
What we are doing here is –
driver.switchTo().defaultContent();
:
First we are switching to the default top most container using the above Selenium code statement.
driver.switchTo().frame(0);
:
This line uses the switchTo()
method of the WebDriver to switch the focus of the driver to the frame
at index 0. Indexing of frames starts from 0. This means that subsequent interactions with elements using the WebDriver will be within the context of this frame.
driver.findElement(By.xpath("//input[@placeholder='Username']"));
:
This line finds an element within the current frame. The By.xpath("//input[@placeholder='Username']")
selector is used to locate an element with the attribute placeholder
having the value “Username“.
.sendKeys("LogicalDuniya_User1");
:
This line sends the text “LogicalDuniya_User1” to the located element. This action simulates typing in the input field, which is a username field.
2] Switch to frame using ‘id’ attribute :
If a frame
web element has id
or name
attribute, you can switch to that frame
by providing that attribute value. Here is a simple example with HTML Source code and Selenium script –
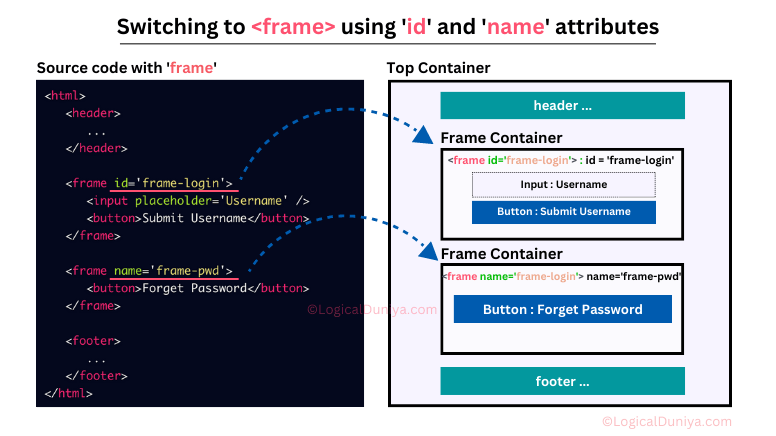
Source Code – HTML
<html>
<header>
...
</header>
<frame id='frame-login'>
<input placeholder='Username' />
<button>Submit Username</button>
</frame>
<frame name='frame-pwd'>
<button>Forget Password</button>
</frame>
<footer>
...
</footer>
</html>
Script Code – To handle frame in Selenium
// Switch to default top container - This is optional statement, if WebDriver object is already focusing to Top Container
driver.switchTo().defaultContent();
// Provide the 'id' attribute value of desired frame-
driver.switchTo().frame("frame-login");
// Then perform operation on Web Elements present inside the current frame -
driver.findElement(By.xpath("//input[@placeholder='Username']"))
.sendKeys("LogicalDuniya_User1");
In above Selenium Script, in statement –
driver.switchTo().defaultContent();
:
Here, we are switching to the default content Or, top most container which contains all the web elements. This is an optional statement, if WebDriver
object is already focusing to the Top Level Container.
driver.switchTo().frame("frame-login");
:
Here, in above statement, in the frame()
method we are providing frame
‘s id
attribute value = “frame-login
” to switch the WebDriver object’s focus on that frame
.
- Perform action after switching to frame :
driver.findElement(..)
:
After switching to frame
web container, we have fetched an input text field using xpath = "//input[@placeholder='Username']"
, to input the username = “LogicalDuniya_User1
“
3] Switch to frame using ‘name’ attribute :
Similar way, you can pass ‘name
‘ attribute value to the frame(..)
method. It will search for the frame
web element having that particular name
attribute, and switch to it.
Then, you can perform operations to web element present inside that frame
.
If we take simple example of above HTML Source Code, then to click Forget Password button, you need to write below Selenium script to handle Frames –
Script Code – To handle frame in Selenium
// Switch to default top container - This is optional statement, if WebDriver object is already focusing to Top Container
driver.switchTo().defaultContent();
// Provide the 'name' attribute value of desired frame-
driver.switchTo().frame("frame-pwd");
// Then perform operation on Web Elements present inside the current frame -
driver.findElement(By.xpath("//button[contains(text(), 'Forget Password')]"))
.click();
Conclusion –
In this simple – pictorial based Selenium tutorial, we learnt about – what the core steps to Handle Frames in Selenium? We have also understood how to use different variants of switch(..)
method such as with frame index
, frame id
and frame name
. Hope, now you have better understanding of handling frame
and iframe
web elements in Selenium.
This in-depth tutorial is part of the Selenium Framework Mastery Series. You can check this link to learn much more interesting topics of Selenium in simple words.
If you have any query related to Selenium, Programming, Resume or Career, then just drop your query in the below comments section, and I’ll try my best to provide answer to your query within 24 hours.
Keep thinking logical . .
Team LogicalDuniya.com
Cheers, Shubham !!
[…] errors related to frame and parent frame. There is another in-depth tutorial explaining – How to handle Frames in Selenium? Also, there is a complete Selenium Framework Mastery Series, in which you will learn a lot of […]
Very helpful post on frames .. Keep posting guys.